ScrollView and HorizontalScrollView are layout container for a view hierarchy that can be scrolled vertically or horizontally by the user, allowing it to be larger than the physical display. A ScrollView/HorizontalScrollView is a FrameLayout, meaning you should place one child in it containing the entire contents to scroll; this child may itself be a layout manager with a complex hierarchy of objects.
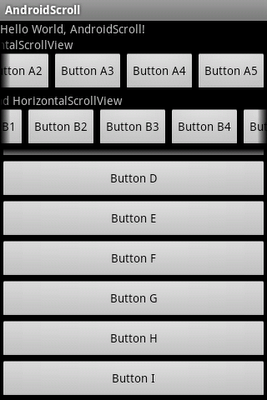
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<HorizontalScrollView
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Inside 1st HorizontalScrollView" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<Button
android:text="Button A1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button A2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button A3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button A4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button A5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
</LinearLayout>
</HorizontalScrollView>
<HorizontalScrollView
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Inside 2nd HorizontalScrollView" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<Button
android:text="Button B1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button B2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button B3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button B4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button B5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<Button
android:text="Button B6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
</LinearLayout>
</HorizontalScrollView>
<ScrollView
android:layout_height="fill_parent"
android:layout_width="fill_parent">
<LinearLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Inside ScrollView" />
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Button C"/>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Button D"/>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Button E"/>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Button F"/>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Button G"/>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Button H"/>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Button I"/>
</LinearLayout>
</ScrollView>
</LinearLayout>
Thx
ReplyDeletethank you, this helped me alot!
ReplyDeletethankyou very much
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThank you so much.
ReplyDeleteThat's exactly what i'm looking for.
Silvius
Good day
ReplyDeleteThis something that I was looking for. Very nice, thank you. I have a question, how can we scroll horizontal to another layout is it possible? Thanks
Thx ! It helped me a lot! Good example !
ReplyDeletethanks dear....
ReplyDeleteHelpfull tutorial, thanks in advance..
ReplyDeleteThis comment has been removed by the author.
ReplyDeletethank u so much. will u please post the java code for above xml.
ReplyDeleteNo special java code needed.
DeleteYou refer it as normal:
- add android:id="@+id/xxx" in xml.
- findViewById(R.id.xxx) in Java.
This comment has been removed by the author.
Deletethank u very much my dear friend.it's working now
Deletei have another problem please clarify this one also.the problem is i need to get the image from sdcard and create animation frame using that image. i post my java code and xml code please clear the error or post your own logic code. please friend clear my doubt
my java code:
import java.io.File;
import java.util.ArrayList;
import android.app.Activity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.drawable.AnimationDrawable;
import android.graphics.drawable.BitmapDrawable;
import android.os.Bundle;
import android.os.Environment;
import android.widget.ImageView;
public class FixActivity extends Activity {
/** Called when the activity is first created. */
ImageView image1;
AnimationDrawable animate;
BitmapDrawable bit1,bit2,bit3,bit4;
ArrayList bitmapArray;
File file;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
file = new File(Environment.getExternalStorageDirectory()+"/Pictures/img");
File imageList[] = file.listFiles();
image1=(ImageView)findViewById(R.id.imageView1);
for(int i=0;i();
bitmapArray.add(b);
}
bit1 =new BitmapDrawable(getResources(), bitmapArray.get(0));
bit2 =new BitmapDrawable(getResources(), bitmapArray.get(1));
bit3 =new BitmapDrawable(getResources(), bitmapArray.get(2));
bit4 =new BitmapDrawable(getResources(), bitmapArray.get(3));
int duration1=300;
animate=new AnimationDrawable();
animate.addFrame(bit1, duration1);
animate.addFrame(bit2, duration1);
animate.addFrame(bit3, duration1);
animate.addFrame(bit4, duration1);
animate.setOneShot(true);
image1.setBackgroundDrawable(animate);
animate.setVisible(true, false);
animate.start();
}
}
good and superb example code dude,give some more on others also.
ReplyDeletecan i know, how can i add the new image dynamically in the HorizontalScrollView
ReplyDeletePlease read Insert ImageView dynamically using Java code.
Delete