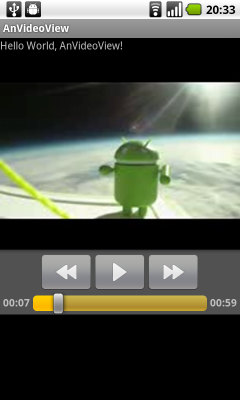
main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> <LinearLayout android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="wrap_content"> <VideoView android:id="@+id/myvideoview" android:layout_width="fill_parent" android:layout_height="wrap_content" /> </LinearLayout> </LinearLayout>
package com.AnVideoView; import android.app.Activity; import android.net.Uri; import android.os.Bundle; import android.widget.MediaController; import android.widget.VideoView; public class AnVideoView extends Activity { String SrcPath = "rtsp://v5.cache1.c.youtube.com/CjYLENy73wIaLQnhycnrJQ8qmRMYESARFEIJbXYtZ29vZ2xlSARSBXdhdGNoYPj_hYjnq6uUTQw=/0/0/0/video.3gp"; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); VideoView myVideoView = (VideoView)findViewById(R.id.myvideoview); myVideoView.setVideoURI(Uri.parse(SrcPath)); myVideoView.setMediaController(new MediaController(this)); myVideoView.requestFocus(); myVideoView.start(); } }
Related Post:
- Using VideoView to play mp4 from sdcard
- Dual VideoView to play 3gp from YouTube
- How to get 3gp link of Youtube video
This example cannot be run on emulator! You need a true device to run it.
ReplyDeleteBcoz Emulator,does not support online streaming facilities.its a pureally virtual machine.so try in and Android mobile or tablets
DeleteThanks Eric for the example!
ReplyDeleteDo you now how to show the MediaController when starting the Activity so that the user can see how long is the video and where the control buttons are?
I have tried many things but did not managed to do that so far.
Thanks,
BJ
BJ,
ReplyDeleteSorry, I can't catch your question - The example have already show the play time and controls.
Hi i m getting an msg that "sorry this video cannot be played".please help ASAP
ReplyDeleteHey,but you trying to open that video in Emulator,or android phone?
Deletei trying already in android phone but cannot play 3gp video in my device.
DeletePRABHJOT SINGH,
ReplyDeleteDo you run on emulator? or un-supported format?
same problem, I've run it on device
ReplyDeleteHello guys! I copy this code into my empty project, but it doesn't work (( It said me that video loagind is impossible. Help me please. I try it on my device.
ReplyDeleteMust I edit a AndroidManifest file?
ReplyDeleteYes. go to AndroidManifest. click on Permission Tab.
DeleteChoose Add. Select the Name as android.permission.INTERNET
it allows your app to connect to the internet.
if you load from internet, for sure you have to edit AndroidManifest.
ReplyDeleteit does not works...it says "sorry this video cannot be Played"
ReplyDeleteHello guys, Please let anybody know's about how to play the video using buffer? I have received buffers from network.
ReplyDeleteplease change the path of ur video in plcae of srcpath and add the internet permission line in manifest file thanku
ReplyDeletehi i just started using android and i need to drag a video
ReplyDeletemeans that i can show a video with a big resolution on my android emulator using the keyboards up, left, down and right
please can anyone help me
thanks very much
can you please tell me how can i play two video on screen?give me the full code with xml.please :(
ReplyDeleteHello ,
ReplyDeleteThe reason you guys are getting error "sorry this video cannot be Played" is because you guys are playing the video through the WiFi avilable in the device. Just turn off the WiFi and turn on 3g , Video will be played .
rtsp links are supported in 3G
its not required to turn off WiFi... i tested this code on wifi enabled device (tho i use only Wifi Data on my tab)...its wrking fine...its also supported in 2G (tested)...
Deletetanu:
ReplyDeleteplease read: Dual VideoView to play 3gp from YouTube.
how to live stream ?
ReplyDeleteenkhbayar,
ReplyDeleteI'm not sure. Can you provide any link for example?
How did you get that youtube link?
ReplyDeleteSearch in YouTube mobile site: http://m.youtube.com/
Deletepourquoi la lecture vidéo n'est pas d'une bonne qualité et est ce que la capture vidéo fonctionne sur l'émulateur car j'ai testé plusieurs tuto mai il ne fonctionnent pas et la taille du fichier est toujour égale à 0
ReplyDeleteI get an error saying Video cannot be played ? How do I fix this error ? What changes need to be made to the android manifest ? I already have internet permissions enabled for my application.
ReplyDeleteSome case is it cannot be downloaded, or the format is not supported.
DeleteIf you have any query realted android or video file not supported,or video cant play.so mail me your query asap.i can solve ..
ReplyDeleteprashant.nikam.ps@gmail.com
ReplyDeleteWhat to do if teh url is not in the 3gp format,for example if the url is like http://www.youtube.com/watch?v=jvcNOz1NHKM
ReplyDeleteSuggest to play 3gp version in this case.
DeleteYou can search your 3gp version in http://m.youtube.com/
The url of your 3gp version is :
rtsp://v7.cache6.c.youtube.com/CjYLENy73wIaLQmjHE09Ow33jhMYDSANFEIJbXYtZ29vZ2xlSARSBXdhdGNoYKjR78WV1ZH5Tgw=/0/0/0/video.3gp
How can i get the 3gp version of a link after i search for something? so that the player should be dinamically
DeleteIf you know this, please help, my email: rosu_alin@ymail.com
DeletePlease read Get 3gp link of Youtube video.
Deletehey,i have done my project successfully show on the emulator but video is not running on that and error like:
ReplyDeletesorry this video can not be played
Hello,
ReplyDeleteIs it possible to run the youtube video using Android Native Media Player? Also would like to add that can we buffer/cache the video in background and user can run it afterwards?
Pleas read Play Youtube 3gp video with MediaPlayer in SurfaceView.
ReplyDeleteHello please help me. i just want to play youtube video as your code reference . i can play your video 3gp link its good. but however i put my url in string path it always says "sorry this video can't be play". this message for all video url. i have learn how to get video url :"right click on video url and copy it." but then not success . i run this in real device . what is the problem ?
ReplyDeletefor those who got the message "sorry this video can't be play":
ReplyDeleteEdit android manifest.xml:
can you use with youtube api bro :)
ReplyDeletehow to show loading/buffering of video play from URL before loading...........
ReplyDeleteHi I want to create app for Android Smart TV Box(Minix) and i want to play RTMP or HLS RTSP(mp4) streaming video, please help me How can I do that,.
ReplyDeleteI tried with ur code but am getting this error "Sorry this video cant be played" how can i resolve it,.please help me,.
Thank you.
Please check if your video is Android Supported Media Formats
Deleteyes its a mp4 but rtmp stream,.
DeleteI think Android build-in player not support rtmp stream!
DeleteYa it is, but am using vitamio player to play my stream its perfectly working in simulator but on device (Android Box- minix) its not working also gives this error "This video can't be played" and this is my url (rtmp://fml.8888.planetstream.net/208888/newry).
DeleteThank you.
I to run the app I get an error message "Sorry, you can not play this video"
ReplyDeleteBonjour, j’ai besoin de retourner à la page d’accueil de mon application à la fin de la lecture de la vidéo!
ReplyDeletej’éspère que vous pouvez m’aider, merci d’avance
I have added same code in my application, and also added Internet, wifi and network permissions in manifest.xml file. . I have parsed rtsp url. After running App in emulator I checked logcat, it gives "Couldn't open file on client side, trying server side". But I thought this is because I tried running on emulator then tested on android device, but the problem is same, "Sorry, you can not play this video". Can anyone please help me.?
ReplyDelete