It's a example of Android app to generate QR Code using Google Chart Tools APIs.
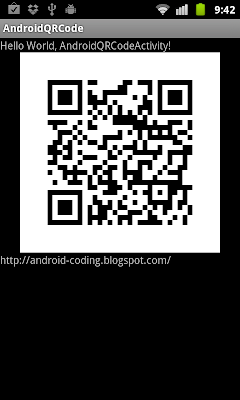
package com.AndroidQRCode;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLConnection;
import android.app.Activity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
public class AndroidQRCodeActivity extends Activity {
final static String urlGoogleChart = "http://chart.apis.google.com/chart";
final static String urlQRApi = "?chs=400x400&cht=qr&chl=";
final static String urlMySite = "http://android-coding.blogspot.com/";
ImageView QRCode;
TextView MySite;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
QRCode = (ImageView)findViewById(R.id.qrimage);
MySite = (TextView)findViewById(R.id.mysite);
MySite.setText(urlMySite);
Bitmap bm = loadQRCode();
if(bm == null){
Toast.makeText(AndroidQRCodeActivity.this,
"Problem in loading QR Code1",
Toast.LENGTH_LONG).show();
}else{
QRCode.setImageBitmap(bm);
}
}
private Bitmap loadQRCode(){
Bitmap bmQR = null;
InputStream inputStream = null;
try {
inputStream = OpenHttpConnection(urlGoogleChart + urlQRApi + urlMySite);
bmQR = BitmapFactory.decodeStream(inputStream);
inputStream.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return bmQR;
}
private InputStream OpenHttpConnection(String strURL) throws IOException{
InputStream is = null;
URL url = new URL(strURL);
URLConnection urlConnection = url.openConnection();
try{
HttpURLConnection httpConn = (HttpURLConnection)urlConnection;
httpConn.setRequestMethod("GET");
httpConn.connect();
if (httpConn.getResponseCode() == HttpURLConnection.HTTP_OK) {
is = httpConn.getInputStream();
}
}catch (Exception ex){
}
return is;
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<ImageView
android:id="@+id/qrimage"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/mysite"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
Permission: "android.permission.INTERNET" is needed.
Related Post:
- Generate 3D Pie Chart using Google Chart Tools
The Google Chart Tools: Inforgraphics has been deprecated and will not longer be supported after 2015. What will happen to QR Codes that have been generated by Google Chart Tools after it is no longer supported? I know that the URL (https://chart.googleapis.com/chart?) will no longer return a generated QR Code. But what about QR Codes that were generated to go to a URL and then the QR image file was saved? Will those QR Codes still go to the URL if scanned after the full deprecation of Google Chart Tools?
ReplyDeleteandroid qrcode generate library