It's a simple light meter Using Android device build-in light sensor. A ProgressBar is defined in main.xml as a indicator of the light sensor. The default value of 100 defined in android:max is a dummy value, it will be updated after lightSensor.getMaximumRange(). The updated reading will be be read in lightSensorEventListener, and update the ProgressBar accordingly.
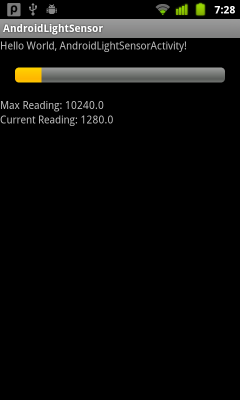
package com.AndroidLightSensor;
import android.app.Activity;
import android.content.Context;
import android.hardware.Sensor;
import android.hardware.SensorEvent;
import android.hardware.SensorEventListener;
import android.hardware.SensorManager;
import android.os.Bundle;
import android.widget.ProgressBar;
import android.widget.TextView;
import android.widget.Toast;
public class AndroidLightSensorActivity extends Activity {
ProgressBar lightMeter;
TextView textMax, textReading;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
lightMeter = (ProgressBar)findViewById(R.id.lightmeter);
textMax = (TextView)findViewById(R.id.max);
textReading = (TextView)findViewById(R.id.reading);
SensorManager sensorManager
= (SensorManager)getSystemService(Context.SENSOR_SERVICE);
Sensor lightSensor
= sensorManager.getDefaultSensor(Sensor.TYPE_LIGHT);
if (lightSensor == null){
Toast.makeText(AndroidLightSensorActivity.this,
"No Light Sensor! quit-",
Toast.LENGTH_LONG).show();
}else{
float max = lightSensor.getMaximumRange();
lightMeter.setMax((int)max);
textMax.setText("Max Reading: " + String.valueOf(max));
sensorManager.registerListener(lightSensorEventListener,
lightSensor,
SensorManager.SENSOR_DELAY_NORMAL);
}
}
SensorEventListener lightSensorEventListener
= new SensorEventListener(){
@Override
public void onAccuracyChanged(Sensor sensor, int accuracy) {
// TODO Auto-generated method stub
}
@Override
public void onSensorChanged(SensorEvent event) {
// TODO Auto-generated method stub
if(event.sensor.getType()==Sensor.TYPE_LIGHT){
float currentReading = event.values[0];
lightMeter.setProgress((int)currentReading);
textReading.setText("Current Reading: " + String.valueOf(currentReading));
}
}
};
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<ProgressBar
android:id="@+id/lightmeter"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:padding="20dp"
style="?android:attr/progressBarStyleHorizontal"
android:max="100"
android:progress="0"
/>
<TextView
android:id="@+id/max"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/reading"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
Thankyou, Its working fine
ReplyDelete