In previoud posts, we have created a custom SurfaceView(MyGameSurfaceView.java) with background thread. Now we are going to create another MyForeground class by extending our MyGameSurfaceView, with overriding code. Base on the existing MyGameSurfaceView class, it's easy to implement the new MyForeground class.

- Create a new class: Right click to select our package (com.MyGame) in Package Explorer, select File -> New -> Class in Eclipse Menu.

- Enter class name, MyForeground, in Name field. And click Browse...
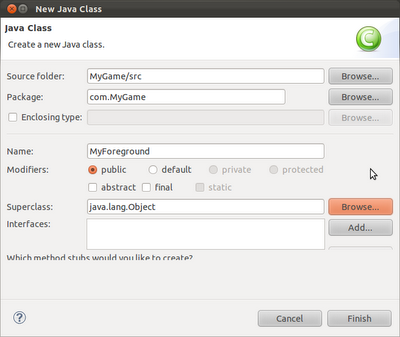
- Type MyGameSurfaceView in Choose a type box, and select MyGameSurfaceView - com.MyGame, click OK.

- Scroll down in New Java Class dialog, click to check Constructors from superclass. Click Finish.

- Now, we have a new class MyForeground.java under com.MyGame.

- Modify MyForeground.java to override onDraw(Canvas canvas). We are going to draw each pixel on foreground, so we need not to handle the "Flickering problems". For now, we simple draw a icon on a fixed location on foreground.
package com.MyGame;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.util.AttributeSet;
public class MyForeground extends MyGameSurfaceView {
public MyForeground(Context context) {
super(context);
// TODO Auto-generated constructor stub
}
public MyForeground(Context context, AttributeSet attrs) {
super(context, attrs);
// TODO Auto-generated constructor stub
}
public MyForeground(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
// TODO Auto-generated constructor stub
}
@Override
protected void onDraw(Canvas canvas) {
Bitmap me = BitmapFactory.decodeResource(getResources(), R.drawable.icon_me);
int loc_x = canvas.getWidth()/2;
int loc_y = canvas.getHeight()/2;
canvas.drawBitmap(me, loc_x, loc_y, null);
}
}
- Modify main.xml to add a new MyForeground, overlape with original MyGameSurfaceView inside a FrameLayout.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<FrameLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<com.MyGame.MyGameSurfaceView
android:id="@+id/myview1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" />
<com.MyGame.MyForeground
android:id="@+id/myforeground"
android:layout_width="fill_parent"
android:layout_height="fill_parent" />
</FrameLayout>
</LinearLayout>
- Modify MyGameActivity.java to handle MyForeground. Please note that in order to make the MyForeground transparency, we have to add the code:
myForeground.setZOrderOnTop(true);
myForeground.getHolder().setFormat(PixelFormat.TRANSPARENT);
package com.MyGame;
import android.app.Activity;
import android.graphics.PixelFormat;
import android.os.Bundle;
public class MyGameActivity extends Activity {
MyGameSurfaceView myGameSurfaceView1;
MyForeground myForeground;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
myGameSurfaceView1 = (MyGameSurfaceView)findViewById(R.id.myview1);
myForeground = (MyForeground)findViewById(R.id.myforeground);
//Set myForeground using transparent background
myForeground.setZOrderOnTop(true);
myForeground.getHolder().setFormat(PixelFormat.TRANSPARENT);
}
@Override
protected void onResume() {
// TODO Auto-generated method stub
super.onResume();
myGameSurfaceView1.MyGameSurfaceView_OnResume();
myForeground.MyGameSurfaceView_OnResume();
}
@Override
protected void onPause() {
// TODO Auto-generated method stub
super.onPause();
myGameSurfaceView1.MyGameSurfaceView_OnPause();
myForeground.MyGameSurfaceView_OnPause();
}
}
No comments:
Post a Comment