The methods onSaveInstanceState(Bundle outState) and onRestoreInstanceState(Bundle savedInstanceState) are the good place to Save and Restore Instance State.
- onSaveInstanceState (Bundle outState)
Called to retrieve per-instance state from an activity before being killed so that the state can be restored in onCreate(Bundle) or onRestoreInstanceState(Bundle) (the Bundle populated by this method will be passed to both).
This method is called before an activity may be killed so that when it comes back some time in the future it can restore its state. For example, if activity B is launched in front of activity A, and at some point activity A is killed to reclaim resources, activity A will have a chance to save the current state of its user interface via this method so that when the user returns to activity A, the state of the user interface can be restored via onCreate(Bundle) or onRestoreInstanceState(Bundle).
Do not confuse this method with activity lifecycle callbacks such as onPause(), which is always called when an activity is being placed in the background or on its way to destruction, or onStop() which is called before destruction. One example of when onPause() and onStop() is called and not this method is when a user navigates back from activity B to activity A: there is no need to call onSaveInstanceState(Bundle) on B because that particular instance will never be restored, so the system avoids calling it. An example when onPause() is called and not onSaveInstanceState(Bundle) is when activity B is launched in front of activity A: the system may avoid calling onSaveInstanceState(Bundle) on activity A if it isn't killed during the lifetime of B since the state of the user interface of A will stay intact.
The default implementation takes care of most of the UI per-instance state for you by calling onSaveInstanceState() on each view in the hierarchy that has an id, and by saving the id of the currently focused view (all of which is restored by the default implementation of onRestoreInstanceState(Bundle)). If you override this method to save additional information not captured by each individual view, you will likely want to call through to the default implementation, otherwise be prepared to save all of the state of each view yourself.
If called, this method will occur before onStop(). There are no guarantees about whether it will occur before or after onPause().
- onRestoreInstanceState (Bundle savedInstanceState)
This method is called after onStart() when the activity is being re-initialized from a previously saved state, given here in savedInstanceState. Most implementations will simply use onCreate(Bundle) to restore their state, but it is sometimes convenient to do it here after all of the initialization has been done or to allow subclasses to decide whether to use your default implementation. The default implementation of this method performs a restore of any view state that had previously been frozen by onSaveInstanceState(Bundle).
This method is called between onStart() and onPostCreate(Bundle).
Example:
package com.example.androidsavestate;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
TextView textviewSavedState;
EditText edittextEditState;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textviewSavedState = (TextView)findViewById(R.id.savedstate);
edittextEditState = (EditText)findViewById(R.id.editstate);
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onRestoreInstanceState(savedInstanceState);
String stateSaved = savedInstanceState.getString("saved_state");
if(stateSaved == null){
Toast.makeText(MainActivity.this,
"onRestoreInstanceState:\n" +
"NO state saved!",
Toast.LENGTH_LONG).show();
}else{
Toast.makeText(MainActivity.this,
"onRestoreInstanceState:\n" +
"saved state = " + stateSaved,
Toast.LENGTH_LONG).show();
textviewSavedState.setText(stateSaved);
edittextEditState.setText(stateSaved);
}
}
@Override
protected void onSaveInstanceState(Bundle outState) {
// TODO Auto-generated method stub
super.onSaveInstanceState(outState);
String stateToSave = edittextEditState.getText().toString();
outState.putString("saved_state", stateToSave);
Toast.makeText(MainActivity.this,
"onSaveInstanceState:\n" +
"saved_state = " + stateToSave,
Toast.LENGTH_LONG).show();
}
}
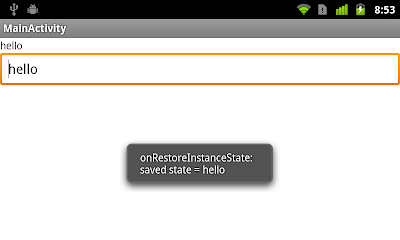
Lovely Blog .....Heads off to you man
ReplyDelete