Involve our comparator on files by calling Arrays.sort(files, filecomparator).
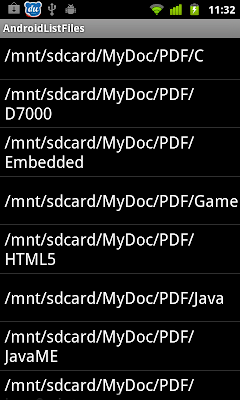
Modify from last post Back in history for File Explorer.
package com.AndroidListFiles;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import java.util.Stack;
import android.app.ListActivity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.view.View;
import android.webkit.MimeTypeMap;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.Toast;
public class AndroidListFilesActivity extends ListActivity {
private List<String> fileList = new ArrayList<String>();
Stack<File> history;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
history = new Stack<File>();
File root = new File(Environment
.getExternalStorageDirectory()
.getAbsolutePath());
ListDir(root);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
// TODO Auto-generated method stub
File selected = new File(fileList.get(position));
if(selected.isDirectory()){
ListDir(selected);
}else {
Uri selectedUri = Uri.fromFile(selected);
String fileExtension
= MimeTypeMap.getFileExtensionFromUrl(selectedUri.toString());
String mimeType
= MimeTypeMap.getSingleton().getMimeTypeFromExtension(fileExtension);
Toast.makeText(AndroidListFilesActivity.this,
"FileExtension: " + fileExtension + "\n" +
"MimeType: " + mimeType,
Toast.LENGTH_LONG).show();
//Start Activity to view the selected file
Intent intent;
intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(selectedUri, mimeType);
startActivity(intent);
}
}
void ListDir(File f){
history.push(f);
File[] files = f.listFiles();
Arrays.sort(files, filecomparator);
fileList.clear();
for (File file : files){
fileList.add(file.getPath());
}
ArrayAdapter<String> directoryList
= new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1, fileList);
setListAdapter(directoryList);
}
Comparator<? super File> filecomparator = new Comparator<File>(){
public int compare(File file1, File file2) {
return String.valueOf(file1.getName()).compareTo(file2.getName());
}
};
@Override
public void onBackPressed() {
// TODO Auto-generated method stub
history.pop(); //remove current directory
if(history.empty()){
super.onBackPressed();
}else{
File selected = history.pop(); //Get the File on Top of history
ListDir(selected);
}
}
}
hii ive tried sorting using the above code by using a button but it doesnt work wen i click on the sort bttn plz hlp..
ReplyDeletepublic void addListenerOnSorting() {
final ArrayAdapter directoryList
= new ArrayAdapter(this, android.R.layout.simple_list_item_1, fileList);
setListAdapter(directoryList);
sorting=(Button) findViewById(R.id.sort);
sorting.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Arrays.sort(fifos, filecomparator);
directoryList.notifyDataSetChanged();
}
Comparator filecomparator = new Comparator(){
public int compare(File file1, File file2) {
return String.valueOf(file1.getName()).compareTo(file2.getName());
}
};
});
It seem that you sort fifos, not fileList.
ReplyDeleteso u mean i should be writing Arrays.sort(fileList, filecomaprator)???
Deletebut i have already declared fifos=files in my code..
The method sort(T[], Comparator) in the type Arrays is not applicable for the arguments (List, Comparator)
ReplyDeletei get this error if i write arrays.sort(fileList, filecomparator) :( i have removed fifos=files.