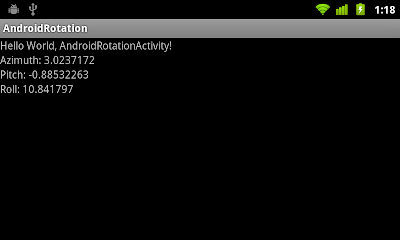
package com.AndroidRotation;
import android.app.Activity;
import android.hardware.Sensor;
import android.hardware.SensorEvent;
import android.hardware.SensorEventListener;
import android.hardware.SensorManager;
import android.os.Bundle;
import android.widget.TextView;
public class AndroidRotationActivity extends Activity implements SensorEventListener{
private SensorManager manager;
private Sensor sensor;
TextView azimuth, pitch, roll;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
manager = (SensorManager)getSystemService(SENSOR_SERVICE);
sensor = manager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER);
azimuth = (TextView)findViewById(R.id.azimuth);
pitch = (TextView)findViewById(R.id.pitch);
roll = (TextView)findViewById(R.id.roll);
}
@Override
protected void onPause() {
super.onPause();
manager.unregisterListener(this);
}
@Override
protected void onResume() {
super.onResume();
manager.registerListener(this, sensor, SensorManager.SENSOR_DELAY_NORMAL);
}
@Override
public void onAccuracyChanged(Sensor arg0, int arg1) {
// TODO Auto-generated method stub
}
@Override
public void onSensorChanged(SensorEvent arg0) {
azimuth.setText("Azimuth: " + String.valueOf(arg0.values[0]));
pitch.setText("Pitch: " + String.valueOf(arg0.values[1]));
roll.setText("Roll: " + String.valueOf(arg0.values[2]));
}
}
Next:
- Get detail info of Accelerometer
Related:
- Detect Orientation using Accelerometer and Magnetic Field sensors