To know more about the project OpenCellID, refer to last post.
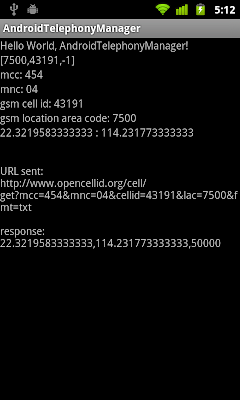
We need the following permission in this example:
- android.permission.ACCESS_COARSE_LOCATION
- android.permission.ACCESS_FINE_LOCATION
- android.permission.READ_PHONE_STATE
- android.permission.INTERNET
package com.AndroidTelephonyManager;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.util.EntityUtils;
import android.app.Activity;
import android.content.Context;
import android.os.Bundle;
import android.telephony.TelephonyManager;
import android.telephony.gsm.GsmCellLocation;
import android.widget.TextView;
public class AndroidTelephonyManager extends Activity {
public class OpenCellID {
String mcc; //Mobile Country Code
String mnc; //mobile network code
String cellid; //Cell ID
String lac; //Location Area Code
Boolean error;
String strURLSent;
String GetOpenCellID_fullresult;
String latitude;
String longitude;
public Boolean isError(){
return error;
}
public void setMcc(String value){
mcc = value;
}
public void setMnc(String value){
mnc = value;
}
public void setCallID(int value){
cellid = String.valueOf(value);
}
public void setCallLac(int value){
lac = String.valueOf(value);
}
public String getLocation(){
return(latitude + " : " + longitude);
}
public void groupURLSent(){
strURLSent =
"http://www.opencellid.org/cell/get?mcc=" + mcc
+"&mnc=" + mnc
+"&cellid=" + cellid
+"&lac=" + lac
+"&fmt=txt";
}
public String getstrURLSent(){
return strURLSent;
}
public String getGetOpenCellID_fullresult(){
return GetOpenCellID_fullresult;
}
public void GetOpenCellID() throws Exception {
groupURLSent();
HttpClient client = new DefaultHttpClient();
HttpGet request = new HttpGet(strURLSent);
HttpResponse response = client.execute(request);
GetOpenCellID_fullresult = EntityUtils.toString(response.getEntity());
spliteResult();
}
private void spliteResult(){
if(GetOpenCellID_fullresult.equalsIgnoreCase("err")){
error = true;
}else{
error = false;
String[] tResult = GetOpenCellID_fullresult.split(",");
latitude = tResult[0];
longitude = tResult[1];
}
}
}
int myLatitude, myLongitude;
OpenCellID openCellID;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
TextView textGsmCellLocation = (TextView)findViewById(R.id.gsmcelllocation);
TextView textMCC = (TextView)findViewById(R.id.mcc);
TextView textMNC = (TextView)findViewById(R.id.mnc);
TextView textCID = (TextView)findViewById(R.id.cid);
TextView textLAC = (TextView)findViewById(R.id.lac);
TextView textGeo = (TextView)findViewById(R.id.geo);
TextView textRemark = (TextView)findViewById(R.id.remark);
//retrieve a reference to an instance of TelephonyManager
TelephonyManager telephonyManager = (TelephonyManager)getSystemService(Context.TELEPHONY_SERVICE);
GsmCellLocation cellLocation = (GsmCellLocation)telephonyManager.getCellLocation();
String networkOperator = telephonyManager.getNetworkOperator();
String mcc = networkOperator.substring(0, 3);
String mnc = networkOperator.substring(3);
textMCC.setText("mcc: " + mcc);
textMNC.setText("mnc: " + mnc);
int cid = cellLocation.getCid();
int lac = cellLocation.getLac();
textGsmCellLocation.setText(cellLocation.toString());
textCID.setText("gsm cell id: " + String.valueOf(cid));
textLAC.setText("gsm location area code: " + String.valueOf(lac));
openCellID = new OpenCellID();
openCellID.setMcc(mcc);
openCellID.setMnc(mnc);
openCellID.setCallID(cid);
openCellID.setCallLac(lac);
try {
openCellID.GetOpenCellID();
if(!openCellID.isError()){
textGeo.setText(openCellID.getLocation());
textRemark.setText( "\n\n"
+ "URL sent: \n" + openCellID.getstrURLSent() + "\n\n"
+ "response: \n" + openCellID.GetOpenCellID_fullresult);
}else{
textGeo.setText("Error");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
textGeo.setText("Exception: " + e.toString());
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<TextView
android:id="@+id/gsmcelllocation"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/mcc"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/mnc"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/cid"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/lac"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/geo"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/remark"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
Related Post:
- OpenCellID
- Convert cellLocation to real location (Latitude and Longitude), from "http://www.google.com/glm/mmap"
- Start Google Maps using intent of ACTION_VIEW
Hi
ReplyDeleteI'm Asraf..
I got the msg "the application has stopped unexpectedly"
Can u pls help me
please post the apk file of this project
ReplyDeletehi
ReplyDeletenice one.i got a error like null pointer exception in this line( int cid = cellLocation.getCid();).
Hello Ramachandran,
DeleteI guess you need to add permission if it told you caused by permission problem.
(I'm in learning this too.)
any one who knows how Geo: uri.parse works, I am using opencellid for long and lat. it works well to open throw uri.parse(view intent) geo:long + lat. But i need to get the url in right way and open in a separately browser
ReplyDeletehello,
Deleteplease check Start Google Maps using intent of ACTION_VIEW.
Thanks Andr
Deletethat is what I do to day, but my intention is to send the Long and lat with sms(texting)to other.
so I need to to know the secret? with Geo: in intent.The Url is probably google.com/glm/mmap but the querystring is unknown for me. When I start google maps using Intent of Action_View i got the right position, but if I take the long and lat and copy n paste it in my browser the position is not the same. I have tried different querystring that I could find ? so I need a solution how to handle it .
Hi,
ReplyDeleteIs it possible to get LAC of the area if MCC,MNC,Lat and Long is known?
If yes, how?
Thanks in advance,
tspshikari
Hi,
ReplyDeleteI got error as
Error: NullPointerException: API key is either null or empty string
can u explain where is the problem
This comment has been removed by the author.
ReplyDeleteIt works but doesn't display the location by giving the following error: android os NetworkOnMainThreadException
ReplyDelete